当我们安装主题和插件时,都会向…
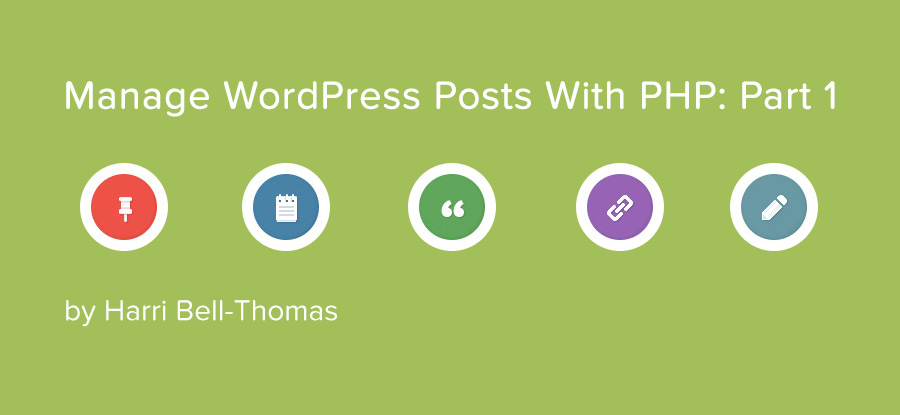
毫无疑问,您已经看到WordPress主题和插件,它们声称在安装它们时会自动为您安装“虚拟数据”,以便您立即拥有一个功能齐全的网站。我将向您展示仅使用PHP函数实现此目标的方法。
如果满足以下条件,这可能会很有用:
- 您的主题或插件需要某些帖子或页面。
- 您要提供如上所述的高级虚拟安装。
- 您想自动创建帖子。
- 您只想学习。
在本教程中,我们将创建一个简单的初学者函数,以实现“快速而肮脏的”工作解决方案。稍后,在另一本教程中,我们将学习如何扩展此处未学到的内容,以创建一个健壮且易于使用的发布系统。
对于那些喜欢使用现有代码而不是阅读所有操作方法的人,这里是我们的最终功能以及其用法和注释示例。
if ( ! function_exists( 'PostCreator' ) ) {
function PostCreator(
$name = 'AUTO POST',
$type = 'post',
$content = 'DUMMY CONTENT',
$category = array(1,2),
$template = NULL,
$author_id = '1',
$status = 'publish'
) {
define( POST_NAME, $name );
define( POST_TYPE, $type );
define( POST_CONTENT, $content );
define( POST_CATEGORY, $category );
define( POST_TEMPLATE, '' );
define( POST_AUTH_ID, $author_id );
define( POST_STATUS, $status );
if ( $type == 'page' ) {
$post = get_page_by_title( POST_NAME, 'OBJECT', $type );
$post_id = $post->ID;
$post_data = get_page( $post_id );
define( POST_TEMPLATE, $template );
} else {
$post = get_page_by_title( POST_NAME, 'OBJECT', $type );
$post_id = $post->ID;
$post_data = get_post( $post_id );
}
function hbt_create_post() {
$post_data = array(
'post_title' => wp_strip_all_tags( POST_NAME ),
'post_content' => POST_CONTENT,
'post_status' => POST_STATUS,
'post_type' => POST_TYPE,
'post_author' => POST_AUTH_ID,
'post_category' => POST_CATEGORY,
'page_template' => POST_TEMPLATE
);
wp_insert_post( $post_data, $error_obj );
}
if ( ! isset( $post ) ) {
add_action( 'admin_init', 'hbt_create_post' );
return $error_obj;
}
}
}
/* All available options for PostCreator()
PostCreator( 'TITLE' , 'POST TYPE' , 'POST CONTENT' , 'POST CATEGORY' , 'TEMPLATE FILE NAME' , 'AUTHOR ID NUMBER' , 'POST STATUS');
TITLE - HTML Stripped Out. Simple String.
POST TYPE - Post type slug. Eg 'post' or 'page'. Custom Post Types are supported.
POST CONTENT - Content of the Post/Page. HTML allowed.
POST CATEGORY - An array of the integer ID's of the category/categories you want to link to your post
TEMPLATE FILE NAME - File name of the template. Only for Pages. In the format 'file_name.php'.
AUTHOR ID NUMBER - Integer value. Default is 1.
POST STATUS - Available options; [ 'draft' | 'publish' | 'pending'| 'future' | 'private' | custom registered status ]
If successful, PostCreator() returns nothing.
If there is an error PostCreator() returns a WP_error object.
*/
PostCreator( 'My Lorem Ipsum', 'page', 'With a sizable serving of Dolor. This was created using Harri Bell-Thomas\'s PostCreator function.' );
逐步指南
我们将创建一个名为PostCreator()的PHP函数,我们将需要它采用某些参数。每个参数都有一个默认值,因此从技术上讲,在调用该函数时,您无需指定任何参数,但是,这有趣之处在哪里?
function PostCreator(
$name = 'AUTO POST',
$type = 'post',
$content = 'DUMMY CONTENT',
$category = array(1,2),
$template = NULL,
$author_id = '1',
$status = 'publish'
) {
// function output here
}
接下来,我将定义一些常量,这些常量对于以下嵌入式函数是必需的。(可以重写为不使用常量,但是我使用了它们,因为我发现它们在扩展基本的PostCreator()函数时很有用,但这是另一个教程的故事。
define( POST_NAME, $name ); define( POST_TYPE, $type ); define( POST_CONTENT, $content ); define( POST_CATEGORY, $category ); define( POST_TEMPLATE, '' ); define( POST_AUTH_ID, $author_id ); define( POST_STATUS, $status );
好的,到目前为止很好。现在,我进行了一些验证,以防止生成重复的帖子/页面(这是一场噩梦,请相信我!)。此验证检查是否已经存在具有相同名称的帖子/页面。如果是这样,它不会创建一个新的,但如果不是,那么它将为您创建一个。
我选择检查帖子标题的原因是因为这就是WordPress生成页面所需的全部(其余部分是自动生成的)。执行此验证的其他方式包括检查“子弹”或帖子ID。所有这些我们都将在以后的教程中介绍。
如果您的插件或主题需要帖子/页面,则此功能特别有用。我首先为我的一个插件开发了它,因为它需要一个带有特定页面模板的页面。使用此功能,我只是保持PostCreator()为WordPress的“ admin_init”,这意味着如果有人尝试删除它(他们敢!),那么它将立即重新创建它,以防止其余的插件出现问题。
请记住,没有人希望他们的博客被劫持,因此请确保您清楚地告诉他们正在发生的事情,并可能为他们提供一个关闭它的选项。
现在回到验证。这是下一部分代码。
if ( $type == 'page' ) { $post = get_page_by_title( POST_NAME, 'OBJECT', $type ); $post_id = $post->ID; $post_data = get_page( $post_id ); define( POST_TEMPLATE, $template ); } else { $post = get_page_by_title( POST_NAME, 'OBJECT', $type ); $post_id = $post->ID; $post_data = get_post( $post_id ); }
那么,这里到底发生了什么?
好吧,本质上是同一过程重复两次。我这样做是因为帖子和页面的处理方式略有不同。此外,仅在尝试创建页面时才定义常量POST_TEMPLATE,因为只有页面可以接受该参数(即,如果您试图创建标准帖子,它将被忽略)。
在IF子句的第一行(如果您还不知道,其技术名称为“ apodosis”),则定义了$ post变量。如果有一个与要创建的名称相同的帖子/页面,则$ post填充现有条目的数据(作为对象,而不是数组,但是如果绝对必要,可以更改)。此变量用于测试标题是否唯一。接下来的两行内容是因为如果要扩展此功能,它们非常有用。例如,更新现有帖子(如果已存在)。
接下来是我们的嵌套函数,它将被添加到“ admin_head”钩子中。这里是;
function hbt_create_post() { $post_data = array( 'post_title' => wp_strip_all_tags( POST_NAME ), 'post_content' => POST_CONTENT, 'post_status' => POST_STATUS, 'post_type' => POST_TYPE, 'post_author' => POST_AUTH_ID, 'post_category' => POST_CATEGORY, 'page_template' => POST_TEMPLATE ); wp_insert_post( $post_data, $error_obj ); }
简而言之,这是使用WordPress的内置函数(wp_insert_post)生成我们的帖子/页面。我们使用我们的参数数组填充$ post_data(您可以在此处看到正在使用的常量)。这将创建,并且如果有错误,它将生成布尔值$ error_obj。TRUE =有问题。FALSE =很好。最后要做的是在管理头上运行前一个功能,但前提是该功能必须通过验证,然后返回错误对象。
if ( ! isset( $post ) ) { add_action( 'admin_init', 'hbt_create_post' ); return $error_obj; }
大!现在,我们创建了很棒的功能,让我们使用它吧!
用法
只需包括PostCreator()函数并运行它。
这将使用默认值运行,但是如果我们要自定义该怎么办?然后我们使用我们的参数。
PostCreator( 'TITLE', 'POST TYPE', 'POST CONTENT', 'POST CATEGORY', 'TEMPLATE FILE NAME', 'AUTHOR ID NUMBER', 'POST STATUS' );
使用所有这些选项时,请注意使用撇号。确保如果要使用单引号(除了参数本身周围的引号之外),请在其前面加上反斜杠。例如;
PostCreator( 'Alex\'s Post' );
TITLE参数接受字符串值。这去除了HTML标记。
例如,POST TYPE参数接受帖子类型的标签。“帖子”或“页面”。支持自定义帖子类型。
PostCreator( 'Alex\'s Post', 'page' );
POST CONTENT’接受一个字符串值。这将是创建的帖子/页面的内容。此处允许HTML。
PostCreator( 'Alex\'s Post', 'page', 'The force is strong with this one…' );
POST CATEGORY接受整数数组。整数对应于归因于帖子/页面的类别的ID。
PostCreator( 'Alex\'s Post', 'page' , 'The force is strong with this one…' , array( 1, 2 ) );
模板文件名是一个字符串值,用于定义新页面的所需页面模板。这仅适用于页面。格式为:’file_name.php’。
PostCreator( 'Alex\'s Post', page', 'The force is strong with this one…', array( 1, 2 ) , 'fullwidth_page.php' );
作者ID编号是作者ID的整数值。
PostCreator( 'Alex\'s Post', 'page', 'The force is strong with this one…', array( 1, 2 ) , 'fullwidth_page.php', '1' );
POST STATUS允许您定义已创建帖子/页面的状态。默认情况下,它是“已发布”。
可用选项; [‘草稿| ‘发布’| “待定” | ‘未来’| “私人” | 自定义注册状态
PostCreator( 'Alex\'s Post', 'page', 'The force is strong with this one…', array( 1, 2 ) , 'fullwidth_page.php', '1', 'publish' );
结论
WordPress是一个非常强大的工具,但有时肯定会很不守规矩。我希望这个简单的代码段对您有所帮助,也许一路上学习一两个东西。请继续关注下一个,我将把本文中已经完成的工作转换为PHP类,从而增加更多的功能和稳定性。要预览,请查看Github上的代码:PostController
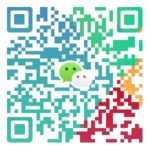
微信扫描二维码联系我们!
我们在微信上24小时期待你的声音
提供外贸路由器设备产品,轻松翻墙,解答:WP主题推荐,WP网站建设,Google SEO,百度SEO,专业服务器环境搭建等!
需要提供WordPress主题/插件的汉化服务可以随时联系我们!另外成品WordPress网站以及半成品WordPress网站建设,海外Google SEO优化托管服务,百度SEO优化托管服务,Centos/Debian服务器WP专用环境搭建,WP缓存服务器搭建,我们都是你的首选,拥有多年WP开源程序服务经验,我们一直在坚持客户体验,没有最好,只有更好!