当我们安装主题和插件时,都会向…
虽然我们是WordPress定制器的忠实支持者,用于添加主题选项,但有些人还是希望为主题创建一个简单的管理面板,或者希望在定制器之外加入基本的管理面板。在本文中,我将向您展示如何使用WordPress本机设置API通过一个简单的类轻松创建自己的管理面板,该类可以轻松扩展以添加更多选项。这不是教程,而是更多的入门代码,可用于创建管理面板,因此在开始之前,您应该了解代码的用法way
创建主题选项面板
以下是可用于创建管理面板的类。该班级将添加一个新的主题选项面板,其中包含3个不同的设置作为示例(复选框,输入和选择)。只需将下面的代码直接插入您的functions.php文件的最底部,或创建一个新文件,然后使用require_once函数将其调用到您的functions.php文件中(首选)。
主题管理页面的屏幕截图
在向您展示代码之前,我想与您分享您将创建的管理面板外观的屏幕截图。
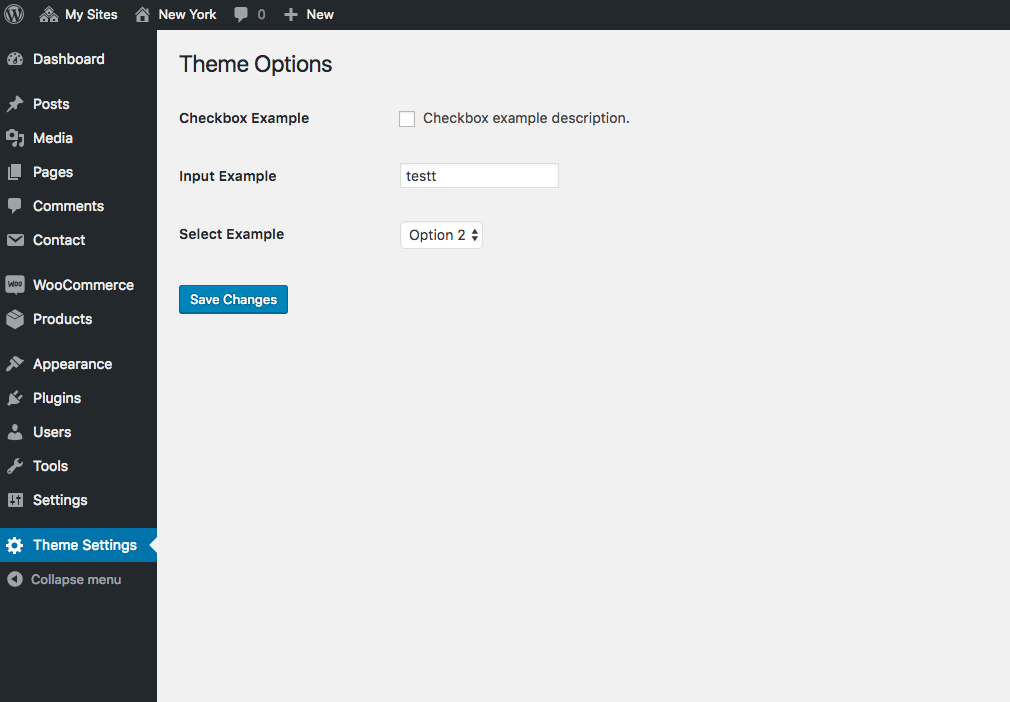
管理员屏幕代码
这是创建管理面板并包含帮助程序功能以获取任何设置的值所需的代码:
<?php /** * Create A Simple Theme Options Panel * */ // Exit if accessed directly if ( ! defined( 'ABSPATH' ) ) { exit; } // Start Class if ( ! class_exists( 'WPEX_Theme_Options' ) ) { class WPEX_Theme_Options { /** * Start things up * * @since 1.0.0 */ public function __construct() { // We only need to register the admin panel on the back-end if ( is_admin() ) { add_action( 'admin_menu', array( 'WPEX_Theme_Options', 'add_admin_menu' ) ); add_action( 'admin_init', array( 'WPEX_Theme_Options', 'register_settings' ) ); } } /** * Returns all theme options * * @since 1.0.0 */ public static function get_theme_options() { return get_option( 'theme_options' ); } /** * Returns single theme option * * @since 1.0.0 */ public static function get_theme_option( $id ) { $options = self::get_theme_options(); if ( isset( $options[$id] ) ) { return $options[$id]; } } /** * Add sub menu page * * @since 1.0.0 */ public static function add_admin_menu() { add_menu_page( esc_html__( 'Theme Settings', 'text-domain' ), esc_html__( 'Theme Settings', 'text-domain' ), 'manage_options', 'theme-settings', array( 'WPEX_Theme_Options', 'create_admin_page' ) ); } /** * Register a setting and its sanitization callback. * * We are only registering 1 setting so we can store all options in a single option as * an array. You could, however, register a new setting for each option * * @since 1.0.0 */ public static function register_settings() { register_setting( 'theme_options', 'theme_options', array( 'WPEX_Theme_Options', 'sanitize' ) ); } /** * Sanitization callback * * @since 1.0.0 */ public static function sanitize( $options ) { // If we have options lets sanitize them if ( $options ) { // Checkbox if ( ! empty( $options['checkbox_example'] ) ) { $options['checkbox_example'] = 'on'; } else { unset( $options['checkbox_example'] ); // Remove from options if not checked } // Input if ( ! empty( $options['input_example'] ) ) { $options['input_example'] = sanitize_text_field( $options['input_example'] ); } else { unset( $options['input_example'] ); // Remove from options if empty } // Select if ( ! empty( $options['select_example'] ) ) { $options['select_example'] = sanitize_text_field( $options['select_example'] ); } } // Return sanitized options return $options; } /** * Settings page output * * @since 1.0.0 */ public static function create_admin_page() { ?> <div class="wrap"> <h1><?php esc_html_e( 'Theme Options', 'text-domain' ); ?></h1> <form method="post" action="options.php"> <?php settings_fields( 'theme_options' ); ?> <table class="form-table wpex-custom-admin-login-table"> <?php // Checkbox example ?> <tr valign="top"> <th scope="row"><?php esc_html_e( 'Checkbox Example', 'text-domain' ); ?></th> <td> <?php $value = self::get_theme_option( 'checkbox_example' ); ?> <input type="checkbox" name="theme_options[checkbox_example]" <?php checked( $value, 'on' ); ?>> <?php esc_html_e( 'Checkbox example description.', 'text-domain' ); ?> </td> </tr> <?php // Text input example ?> <tr valign="top"> <th scope="row"><?php esc_html_e( 'Input Example', 'text-domain' ); ?></th> <td> <?php $value = self::get_theme_option( 'input_example' ); ?> <input type="text" name="theme_options[input_example]" value="<?php echo esc_attr( $value ); ?>"> </td> </tr> <?php // Select example ?> <tr valign="top" class="wpex-custom-admin-screen-background-section"> <th scope="row"><?php esc_html_e( 'Select Example', 'text-domain' ); ?></th> <td> <?php $value = self::get_theme_option( 'select_example' ); ?> <select name="theme_options[select_example]"> <?php $options = array( '1' => esc_html__( 'Option 1', 'text-domain' ), '2' => esc_html__( 'Option 2', 'text-domain' ), '3' => esc_html__( 'Option 3', 'text-domain' ), ); foreach ( $options as $id => $label ) { ?> <option value="<?php echo esc_attr( $id ); ?>" <?php selected( $value, $id, true ); ?>> <?php echo strip_tags( $label ); ?> </option> <?php } ?> </select> </td> </tr> </table> <?php submit_button(); ?> </form> </div><!-- .wrap --> <?php } } } new WPEX_Theme_Options(); // Helper function to use in your theme to return a theme option value function myprefix_get_theme_option( $id = '' ) { return WPEX_Theme_Options::get_theme_option( $id ); }
在主题中使用选项
使用您的选项非常容易,尤其是使用上面代码中包含的辅助功能时。这是一个示例,说明如何使用帮助器函数获取并显示ID为“ select_example”的下拉字段的值。
$value = myprefix_get_theme_option( 'select_example' ); echo $value;
有关创建管理屏幕的更多信息
我看到过大量的管理页面都是以非常不同的方式创建的,但我个人很喜欢上面显示的方法。这非常简单直接。您无需对主题选项如此疯狂!如果您需要更多信息来创建管理屏幕,则需要检出管理屏幕代码。
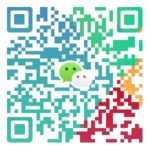
微信扫描二维码联系我们!
我们在微信上24小时期待你的声音
提供外贸路由器设备产品,轻松翻墙,解答:WP主题推荐,WP网站建设,Google SEO,百度SEO,专业服务器环境搭建等!
需要提供WordPress主题/插件的汉化服务可以随时联系我们!另外成品WordPress网站以及半成品WordPress网站建设,海外Google SEO优化托管服务,百度SEO优化托管服务,Centos/Debian服务器WP专用环境搭建,WP缓存服务器搭建,我们都是你的首选,拥有多年WP开源程序服务经验,我们一直在坚持客户体验,没有最好,只有更好!