当我们安装主题和插件时,都会向…
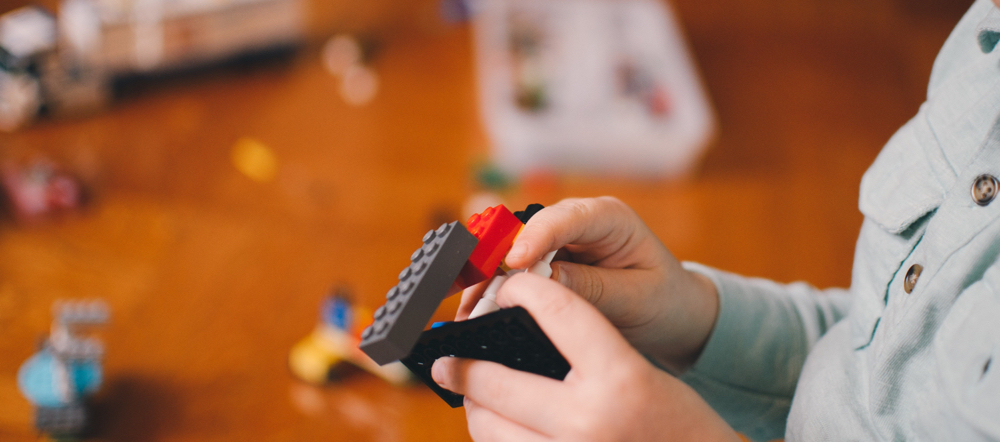
WordPress是一个了不起的内容管理系统,具有许多出色的功能,例如小部件。在本教程中,我将向您解释如何在一个小插件中创建自己的小部件。这篇文章将介绍在创建小部件本身之前您需要了解的一些其他要点。开始了!
步骤1:建立小工具外挂程式
我最近创建了一个名为“ Freelancer Widgets Bundle”的插件,有人问我如何创建这样的插件,因此我决定写这篇文章。第一步是创建插件。如您所见,这不是最困难的部分。插件是激活后会添加到WordPress的额外代码。WordPress通过文件夹创建一个循环,以检索所有可用的插件并在后台将其列出。要创建插件,您需要一个诸如Coda(Mac)或Dreamweaver(PC&Mac)之类的编辑器。我建议您在本地安装的WordPress中创建插件,如果在运行时将其放置在实时服务器上可能会造成一些麻烦。因此,请等待测试我们的插件,然后再将其放置在您的主机上。
现在打开文件夹wp-content/plugins。这是您要添加插件的位置。创建一个新目录并将其命名为“ widget-plugin”(实际上,此名称可以是您想要的任何名称)。即使我们的插件只有一个文件,也最好为每个插件使用一个文件夹。在您的目录中,创建一个名为“ widget-plugin.php”的新文件(此名称也可以更改),然后将其打开。现在,我们要添加第一行代码。WordPress下的插件定义遵循一些规则,您可以在此处在法典上阅读。WordPress定义插件的方法如下:
<?php
/*
Plugin Name: Name Of The Plugin
Plugin URI: http://URI_Of_Page_Describing_Plugin_and_Updates
Description: A brief description of the Plugin.
Version: The Plugin's Version Number, e.g.: 1.0
Author: Name Of The Plugin Author
Author URI: http://URI_Of_The_Plugin_Author
License: A "Slug" license name e.g. GPL2
*/
因此,我们必须修改此代码以使其符合我们的需求:
<?php
/*
Plugin Name: My Widget Plugin
Plugin URI: http://www.wpexplorer.com/create-widget-plugin-wordpress/
Description: This plugin adds a custom widget.
Version: 1.0
Author: AJ Clarke
Author URI: http://www.wpexplorer.com/create-widget-plugin-wordpress/
License: GPL2
*/
保存文件。通过仅将代码添加到我们的widget-plugin.php文件中,我们创建了一个插件!好吧,目前该插件不执行任何操作,但是WordPress可以识别它。为确保确实如此,请进行管理,然后转到“插件”菜单下。如果您的插件出现在插件列表中,则表示您很好,否则请确保您已按照我的说明进行操作,然后重试。您现在可以激活插件。
步骤2:建立小工具
现在,我们将创建小部件本身。该小部件将是扩展核心WordPress类WP_Widget的PHP类。基本上,我们的小部件将通过以下方式定义:
// The widget class class My_Custom_Widget extends WP_Widget { // Main constructor public function __construct() { /* ... */ } // The widget form (for the backend ) public function form( $instance ) { /* ... */ } // Update widget settings public function update( $new_instance, $old_instance ) { /* ... */ } // Display the widget public function widget( $args, $instance ) { /* ... */ } } // Register the widget function my_register_custom_widget() { register_widget( 'My_Custom_Widget' ); } add_action( 'widgets_init', 'my_register_custom_widget' );
这段代码为WordPress提供了系统能够使用小部件所需的所有信息:
- 该构造函数,以启动小部件
- 的 形式()函数 来创建管理的窗口小部件形式
- 该update()函数,拯救小部件编辑期间数据
- 然后使用 widget()函数 在前端显示控件内容
1 –构造函数
构造函数是定义小部件名称和主要参数的代码部分,下面是其外观的示例。
// Main constructor
public function __construct() {
parent::__construct(
'my_custom_widget',
__( 'My Custom Widget', 'text_domain' ),
array(
'customize_selective_refresh' => true,
)
);
}
请不要在小部件名称周围使用__(),WordPress会使用此函数进行翻译。我真的建议您始终使用这些功能,以使您的主题完全可翻译。使用’customize_selective_refresh’参数可以在编辑窗口小部件时在“ 外观”> “自定义”下刷新窗口小部件,因此在进行更改时,仅刷新窗口小部件,而不是刷新整个页面。
2 – form()函数
此功能是在WordPress管理区域(“外观”>“小部件”或“外观”>“自定义”>“小部件”下)创建小部件表单设置的功能。这就是您要输入要在网站上显示的数据的情况。因此,我将说明如何在小部件表单设置中添加文本字段,文本区域,选择框和复选框。
// The widget form (for the backend ) public function form( $instance ) { // Set widget defaults $defaults = array( 'title' => '', 'text' => '', 'textarea' => '', 'checkbox' => '', 'select' => '', ); // Parse current settings with defaults extract( wp_parse_args( ( array ) $instance, $defaults ) ); ?> <?php // Widget Title ?> <p> <label for="<?php echo esc_attr( $this->get_field_id( 'title' ) ); ?>"><?php _e( 'Widget Title', 'text_domain' ); ?></label> <input class="widefat" id="<?php echo esc_attr( $this->get_field_id( 'title' ) ); ?>" name="<?php echo esc_attr( $this->get_field_name( 'title' ) ); ?>" type="text" value="<?php echo esc_attr( $title ); ?>" /> </p> <?php // Text Field ?> <p> <label for="<?php echo esc_attr( $this->get_field_id( 'text' ) ); ?>"><?php _e( 'Text:', 'text_domain' ); ?></label> <input class="widefat" id="<?php echo esc_attr( $this->get_field_id( 'text' ) ); ?>" name="<?php echo esc_attr( $this->get_field_name( 'text' ) ); ?>" type="text" value="<?php echo esc_attr( $text ); ?>" /> </p> <?php // Textarea Field ?> <p> <label for="<?php echo esc_attr( $this->get_field_id( 'textarea' ) ); ?>"><?php _e( 'Textarea:', 'text_domain' ); ?></label> <textarea class="widefat" id="<?php echo esc_attr( $this->get_field_id( 'textarea' ) ); ?>" name="<?php echo esc_attr( $this->get_field_name( 'textarea' ) ); ?>"><?php echo wp_kses_post( $textarea ); ?></textarea> </p> <?php // Checkbox ?> <p> <input id="<?php echo esc_attr( $this->get_field_id( 'checkbox' ) ); ?>" name="<?php echo esc_attr( $this->get_field_name( 'checkbox' ) ); ?>" type="checkbox" value="1" <?php checked( '1', $checkbox ); ?> /> <label for="<?php echo esc_attr( $this->get_field_id( 'checkbox' ) ); ?>"><?php _e( 'Checkbox', 'text_domain' ); ?></label> </p> <?php // Dropdown ?> <p> <label for="<?php echo $this->get_field_id( 'select' ); ?>"><?php _e( 'Select', 'text_domain' ); ?></label> <select name="<?php echo $this->get_field_name( 'select' ); ?>" id="<?php echo $this->get_field_id( 'select' ); ?>" class="widefat"> <?php // Your options array $options = array( '' => __( 'Select', 'text_domain' ), 'option_1' => __( 'Option 1', 'text_domain' ), 'option_2' => __( 'Option 2', 'text_domain' ), 'option_3' => __( 'Option 3', 'text_domain' ), ); // Loop through options and add each one to the select dropdown foreach ( $options as $key => $name ) { echo '<option value="' . esc_attr( $key ) . '" id="' . esc_attr( $key ) . '" '. selected( $select, $key, false ) . '>'. $name . '</option>'; } ?> </select> </p> <?php }
这段代码只需向小部件添加5个字段(标题,文本,文本区域,选择和复选框)。因此,首先为小部件定义默认值,然后下一个函数将使用默认值解析为小部件定义/保存的当前设置(因此,不存在的任何设置都将还原为默认设置,例如您第一次向其添加小部件时您的侧边栏)。最后是每个字段的html。请注意,在添加表单字段时使用了esc_attr(),这样做是出于安全原因。每当您在网站上回显用户定义的变量时,都应确保首先对其进行了清理。
3 – update()函数
update()函数非常简单。当WordPress核心开发人员添加了一个非常强大的小部件API时,我们只需要添加以下代码即可更新每个字段:
// Update widget settings
public function update( $new_instance, $old_instance ) {
$instance = $old_instance;
$instance['title'] = isset( $new_instance['title'] ) ? wp_strip_all_tags( $new_instance['title'] ) : '';
$instance['text'] = isset( $new_instance['text'] ) ? wp_strip_all_tags( $new_instance['text'] ) : '';
$instance['textarea'] = isset( $new_instance['textarea'] ) ? wp_kses_post( $new_instance['textarea'] ) : '';
$instance['checkbox'] = isset( $new_instance['checkbox'] ) ? 1 : false;
$instance['select'] = isset( $new_instance['select'] ) ? wp_strip_all_tags( $new_instance['select'] ) : '';
return $instance;
}
如您所见,我们要做的就是检查每个设置,如果不为空,请将其保存到数据库中。请注意wp_strip_all_tags()和wp_kses_post()函数的使用,这些函数用于在将数据添加到数据库之前对其进行清理。每当您将任何用户提交的内容插入数据库时,都需要确保它没有任何恶意代码。第一个函数wp_strip_all_tags删除除基本文本以外的所有内容,因此您可以将其用于最终值是字符串的任何字段,第二个函数wp_kses_post()是用于帖子内容的相同函数,它删除除基本html之类的链接之外的所有标签,跨度,div,图像等。
4 – widget()函数
widget()函数是一种将在网站上输出内容的函数。这就是您的访客将会看到的。可以对该函数进行自定义以包括CSS类和特定的标签,以完美匹配您的主题显示。这是代码(请不要随意修改此代码以满足您的需求):
// Display the widget public function widget( $args, $instance ) { extract( $args ); // Check the widget options $title = isset( $instance['title'] ) ? apply_filters( 'widget_title', $instance['title'] ) : ''; $text = isset( $instance['text'] ) ? $instance['text'] : ''; $textarea = isset( $instance['textarea'] ) ?$instance['textarea'] : ''; $select = isset( $instance['select'] ) ? $instance['select'] : ''; $checkbox = ! empty( $instance['checkbox'] ) ? $instance['checkbox'] : false; // WordPress core before_widget hook (always include ) echo $before_widget; // Display the widget echo '<div class="widget-text wp_widget_plugin_box">'; // Display widget title if defined if ( $title ) { echo $before_title . $title . $after_title; } // Display text field if ( $text ) { echo '<p>' . $text . '</p>'; } // Display textarea field if ( $textarea ) { echo '<p>' . $textarea . '</p>'; } // Display select field if ( $select ) { echo '<p>' . $select . '</p>'; } // Display something if checkbox is true if ( $checkbox ) { echo '<p>Something awesome</p>'; } echo '</div>'; // WordPress core after_widget hook (always include ) echo $after_widget; }
这段代码并不复杂,所有您需要记住的就是检查是否设置了变量,如果没有设置,并且要打印它,则会收到错误消息。
完整的小部件插件代码
现在,如果您一直正确地遵循您的插件,现在应该可以正常使用了,您可以对其进行自定义以满足您的需求。如果您没有遵循指南,或者想再次检查代码,可以访问Github页面以查看完整代码。
结论
我们看到在插件内部创建窗口小部件非常有趣,现在您必须知道如何创建一个包含具有不同字段类型的窗口小部件的简单插件,并且学习了如何使用高级技术自定义窗口小部件。恭喜,您做得很棒!
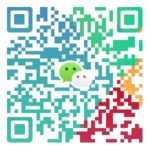
微信扫描二维码联系我们!
我们在微信上24小时期待你的声音
提供外贸路由器设备产品,轻松翻墙,解答:WP主题推荐,WP网站建设,Google SEO,百度SEO,专业服务器环境搭建等!
需要提供WordPress主题/插件的汉化服务可以随时联系我们!另外成品WordPress网站以及半成品WordPress网站建设,海外Google SEO优化托管服务,百度SEO优化托管服务,Centos/Debian服务器WP专用环境搭建,WP缓存服务器搭建,我们都是你的首选,拥有多年WP开源程序服务经验,我们一直在坚持客户体验,没有最好,只有更好!